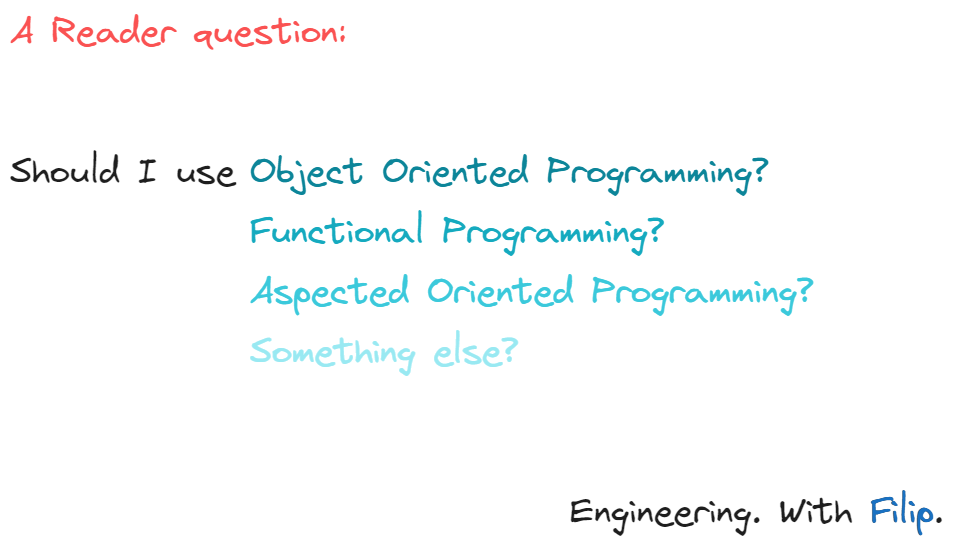
An interesting question popped up in my inbox:
Are there any competitive advantages on using functional programming or programming style over usual programming in .NET API Programming? Advantages in terms of performance and optimisation.
There are two distinct questions here. The first one is about competitive advantages, and the second one is about performance of code.
The answer to the second question is: Not really. The performance benefits we get from choosing one or the other approach is in most cases negligible. What may cause performance degradation is incorrect usage of some technology.
The first question is a bit more tricky, and much more interesting.
What is competitive advantage?
One definition on the internet is:
A competitive advantage is anything that gives a company an edge over its competitors, helping it attract more customers and grow its market share.
Or, to simplify: if a company can deliver it’s services faster, cheaper or with better quality, that company can be considered to have competitive advantage.
What does that have to do with engineering?
Let’s compare the following two pieces of code, which achieve exactly the same result.
Code sample 1:
IEnumerable<int> numbers = ....;
var sum = 0;
foreach (var number in numbers)
{
if (number % 2 == 0)
{
sum += number;
}
}
Code sample 2:
private static int SumEvenNumbers(IEnumerable<int> numbers)
=> numbers
.Where(NumberIsEven)
.Sum();
private static bool NumberIsEven(int arg)
=> arg % 2 == 0;
IEnumerable<int> numbers = ....;
var sum = SumEvenNumbers(numbers);
Which one is easier to read and understand?
Both code samples calculate the sum of all even numbers. Their total complexity is equal. However, code sample 2 is easier to understand just by reading the code, without spending a lot of mental effort to parse and understand what the code does. The name of the function is good enough to give you a pretty good understand of what is it’s responsibility.
This is where FP (Functional programming) or OOP (Object Oriented Programming) shine. They provide good abstractions and allow writing clear and concise code, that reduce cognitive load on developers. That in turn increases development speed and reduces defects. Finally, the business can deliver value faster, and stay ahead of competition.
Takeaway
The takeaway of this article should not be that FP and OOP are good, everything else is bad. Instead, the takeaway is to use any paradigm that allows you to serve the business fast, while keeping the code tidy. In most cases, that would be FP and/or OOP.
Story from the battlefield
I needed to refactor pieces of a project where I knew the domain already. However, I did not know the code base. It took me about a week to have full understanding of what is happening, and which part of the code base is responsible for what. And this was already a small code base of 2-3k lines of code.
After the rewrite, about 80% of code was removed. I managed to do this by introducing objects that represent the domain instead of working with primitives, encapsulating logic and data, having clear method names and have the methods do single thing only.
Then I went ahead and confirmed with a colleague that the code is understandable, even without prior context. In case the business requirements change, we are now able to move fast and deliver value within days, not weeks. And that is competitive advantage.